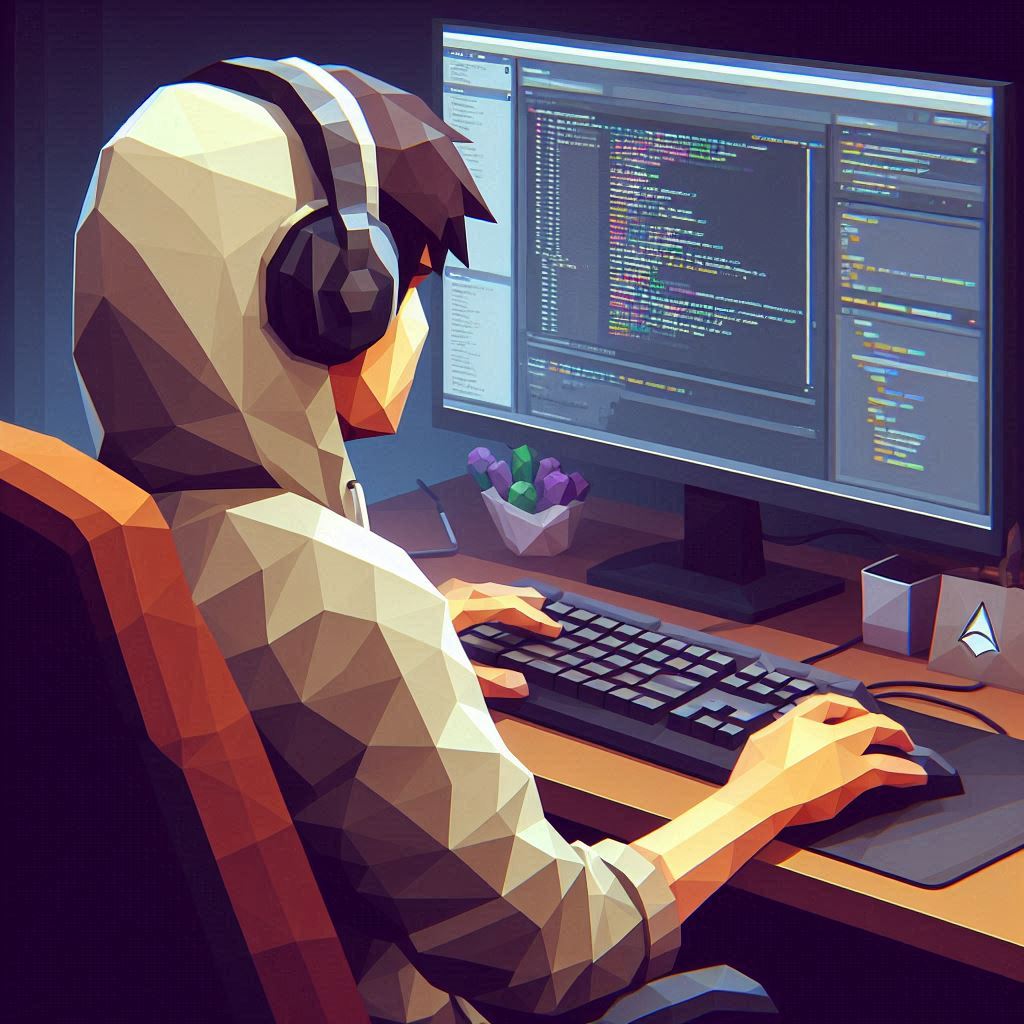
Monke
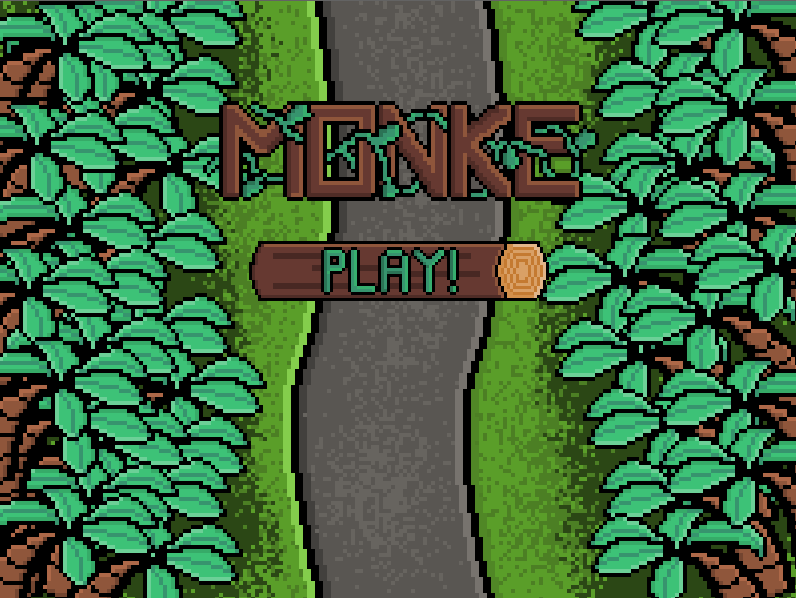
Monke is the first game I have ever made and it was made with the language JavaScript in p5.js. I have made this game for my study, the assignment was to make a version of the game space invaders.
The first thing I created was the player and the player movement. I made this with the “Rect” function. The player movement I made with the “keyisdown” function. I made some limitations for the player with an if statement so it cannot move of the screen.
After I got the player movement figured out I started to create the enemy’s. I made a for loop inside a for loop that creates 3 rows with 7 enemy’s.
After that I made them move from side to side. This was a bit difficult for me because, if one enemy hits the border all of them need to change direction. After some time I figured it out by giving the enemy’s a direction variable.
now that I had enemy’s I thought that it would be handy if the player could shoot them. At first I had made a rectangle called bullet that would always go up. If the bullet would hit the the edge of the screen it would reset at the players posititon. After I made this I had to assign a key so that if the key is pressed a bullet would spawn.
I had this figured out in a short period of time, but you could only shoot one bullet at a time. So I made an array where the bullets will be put in to if the player shoots.
class Bullet {
constructor(X,Y,Width,Height,Speed,Fire,Position,Collision){
this.X = X;
this.Y = Y;
this.Width = Width;
this.Height = Height;
this.Speed = Speed;
this.Fire = Fire;
this.Position = Position;
this.Collision = Collision;
}
//This code makes sure the bullets go up and more bullets at a time
move(){
if(this.Fire == true && this.Position == 0) {
this.Position = 1;
}
if(this.Position == 1) {
this.X = this.X;
this.Y -= this.Speed;
if(this.Y < 0) {
this.Position = 2;
}
}
else {
this.X = player1.X + bulletoffset;
this.Y = player1.Y;
}
for(var i = 0; i < bullets.length; i++){
if(this.Position == 2){
bullets.splice(i,1);
this.Position = 0;
}
}
if(keyCode == 32 && keyIsPressed) {
if(!this.Fire){
this.Fire = true;
} else{
this.Fire = false;
}
}
}
let bullets = [];
function drawBullets() {
for(let i = 0; i < bullets.length; i++){
image(bullet,bullets[i].X,bullets[i].Y,bullets[i].Width,bullets[i].Height)
}
}
After I got the bullets figured out, I began making sure the bullets have collision with the enemy’s. I used a YouTube video to figure this out. I copied the code from the tutorial while listening to the explanation, so it was really easy.
if(this.Collision == true){
this.Position = 2;
this.Collision = false;
}
for(let i = 0;i< aliens.length; i++){
for(let j = 0; j < bullets.length; j++){
if(
bullets[j].X + bullets[j].Width >= aliens[i].X &&
bullets[j].X <= aliens[i].X + aliens[i].Width &&
bullets[j].Y + bullets[j].Height >= aliens[i].Y &&
bullets[j].Y <= aliens[i].Y + aliens[i].Height
) {
bullets[j].Collision = true;
aliens[i].Collision = true;
}
}
}
I also made this for the enemy’s but the bullets go down instead of up. But the thing is, the enemy’s don’t shoot if there is a button pressed. They should be shooting at a time period and I don’t want them to shoot all at once. I made the time period 1 second. After every time period this code will be executed.
let number = Math.floor(Math.random()*aliens.length);
projectiles.push(new Projectile(
aliens[number].X,//X
aliens[number].Y,//y
10,//width
20,//height
5,//speed
false,//collision
0,//position
false//Fire
))
After that I made the shields. The shields are just rectangles with an integer that goes down if they get hit. If the integer is on 0 the shield will dissapear. This was really simple for me.
After the shields I made the “ufo” this is an enemy that goes from left to right at the top of the screen. If you hit it the score that you get is higher than any other enemy. I made this with the math.random function i’ve learned with the enemy projectiles. this was also pretty easy.
After that I made a win and lose condition. it was just a text on the screen.
After that I connected a database to my game. I couldn’t figure this out so I asked a friend to help me and explained everything to me. The code:
const gameID = "9878723611"
const saveDatabase = '__Database_Link__' + 'game='+gameID;
const loadDatabase = '__Database_Link__' + 'game='+gameID;
let topScores = [];
class DatabaseManager{
save(){
httpGet(saveDatabase +'&name='+ entered_name +'&score='+ Score );
console.log("saved")
}
load(){
var loadedData = httpGet(loadDatabase);
loadedData.then(function(result) {
topScores = JSON.parse(result);
topScores.sort(function(a, b){return b.score - a.score});
if (topScores[5] !== undefined) {
topScores.splice(5);
}
})
}
}
const databasemanager = new DatabaseManager ();
After I was done with that I wanted a theme to my game so I thought of monkey’s and gorilla’s. I wanted it to be a bit realistic so I searched for the enemy of a gorilla and it said humans. I made all the sprites for the game with aseprite. For the button on the home screen I made with 5 induvidual sprites that changes for every 5 frames and inverted.
After all that this is the end result: