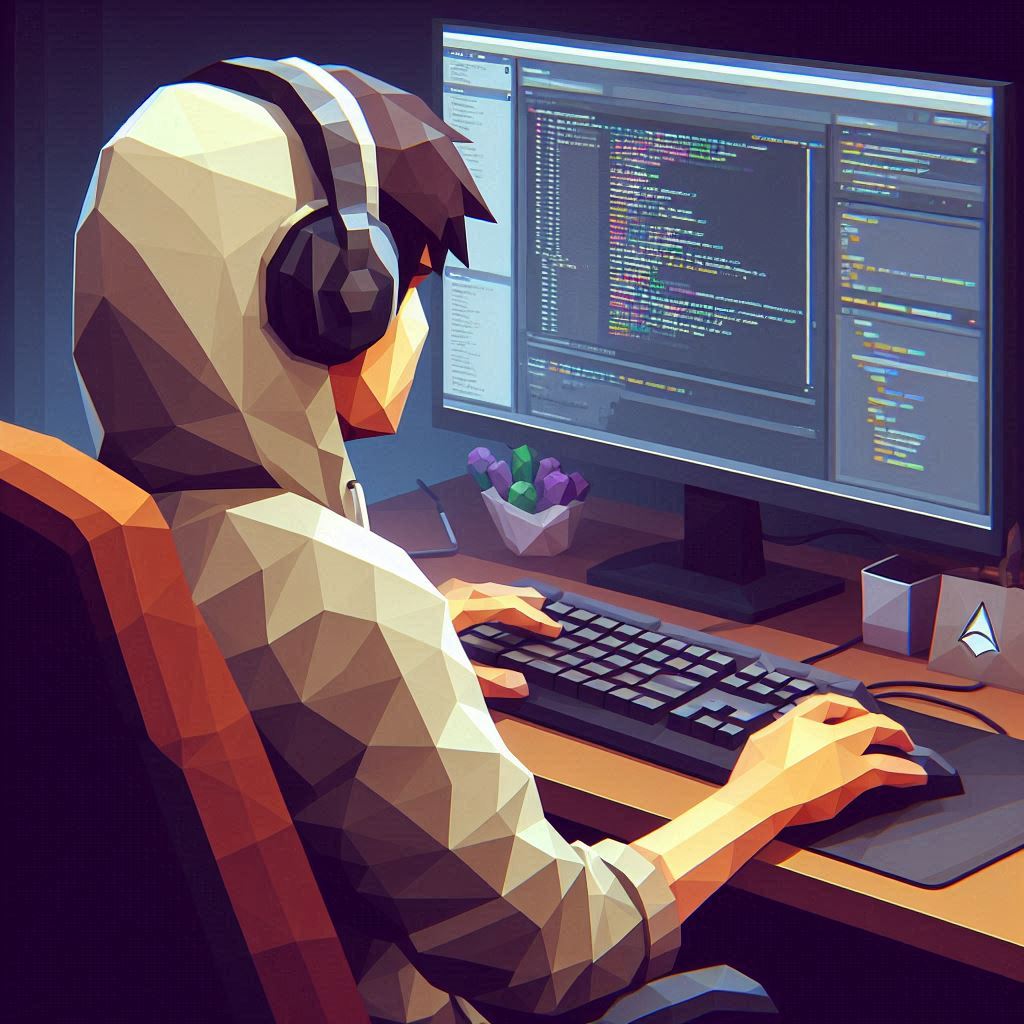
Space evader
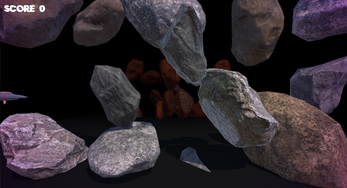
Space evader is the first game I have ever made with Unity. It was supposed to be a flappy bird game in 3D but I made my own twist to it. In this game you are an UFO that wants to travel through space but there are a lot of meteorites in the way. The goal of the game is to beat your own highscore.
The first thing I made was the player. I started with a sphere object that moves on the screen with gravity. I used the Unity Input Manager Package for this so it was pretty easy to code. After that I made it so that the player couldn’t leave the screen. After that I added a lose condition by adding a plane object and if the player hits something there would be a log that says: “Game Over”.
After that I made obstacles that were just walls that would go to the player with holes in them and different colors. It was a bit tricky because I found it hard to change the color of the obstacles. I asked a friend how I could fix this, he said that I was changing the color of the prefab and not the instance that was spawned in the game.
After I made the obstacles, I made the UI. I used the Unity UIToolkit for this because my teacher wanted me to learn to use the UIToolkit. I found it a bit tricky to save the highest score and display it but after reading the site that was recommended at the assignment it was pretty easy. The code:
public void OnGameOver()
{
if(PlayerPrefs.GetInt("HighScore", 0) < player.GetComponent<PlayerStatistics>().score)
{
audioManager.PlaySFX(audioManager.HighScore);
PlayerPrefs.SetInt("HighScore", player.GetComponent<PlayerStatistics>().score);
}
scoreLabel.Clear();
uiDoc.rootVisualElement.Clear();
uiDoc.rootVisualElement.Add(gameoverscreen.CloneTree());
uiButton = uiDoc.rootVisualElement.Q<Button>("RetryButton");
gameOverScoreLabel = uiDoc.rootVisualElement.Q<Label>("YourScore");
highScoreLabel = uiDoc.rootVisualElement.Q<Label>("HighScore");
gameOverScoreLabel.text = "Your Score: " + GameObject.Find("Player").GetComponent<PlayerStatistics>().score;
highScoreLabel.text = "High Score: " + PlayerPrefs.GetInt("HighScore", 0);
uiButton.clicked += OnRetryButtonClicked;
}
After that the assignment I got was: “add models and animations.” This is where the theme of the game came from because I found an UFO model on the internet. After I found the UFO model I wanted to create a space ship flying through the trenches of the star wars death star, but I couldn’t find good models and textures so made a UFO flying through space. I made a meteor controller that every meteor has so it rotates. A nice little effect but makes de game alot more alive and interesting to play through.
public class MeteoriteRotator : MonoBehaviour
{
// Update is called once per frame
void Update()
{
transform.Rotate(new Vector3(15, 30, 45) * Time.deltaTime);
}
}
The Assignment after that was to add sound effects and music, this was pretty easy.
The last assignment was to add post processing so I added a vignette effect, fog effect, depth of field effect, ambient occlusion and anti-aliasing. This was all pretty easy. This is the end result: